
Best AI Development Practices streamline project workflows. They ensure efficiency, accuracy, and innovation in every machine learning and AI initiative.
As AI technology continues to evolve, it is essential to adopt best practices to ensure efficient and accurate development. AI coding best practices, AI development techniques, and AI coding standards must be adhered to when creating AI software applications.
Best AI project practices, AI software development guidelines, AI coding tips are all important aspects to consider in achieving optimal AI development. Additionally, AI project management best practices must be adopted to ensure that projects are managed effectively and efficiently.
AI Development Techniques
Artificial intelligence (AI) development techniques are essential to creating intelligent applications that can recognize patterns and make decisions based on data. There are several techniques used in AI development, including machine learning algorithms, neural networks, and natural language processing.
Machine learning algorithms are used to train AI models by analyzing data and identifying patterns. These algorithms are categorized into three types: supervised learning, unsupervised learning, and reinforcement learning.
Neural networks are a set of algorithms designed to recognize patterns in data. They are used in applications such as image recognition and natural language processing. A neural network consists of layers of interconnected nodes that process and transmit information.
Natural language processing (NLP) is a technique used to enable machines to understand human language. NLP algorithms are used in speech recognition and language translation applications. They use machine learning techniques to analyze and interpret human language.

Code Examples in TensorFlow and PyTorch
Popular AI frameworks like TensorFlow and PyTorch provide built-in support for these techniques, making it easier for developers to implement them. Code examples in both frameworks are provided below to illustrate how these AI techniques can be used in practice.
Technique | TensorFlow Code Example | PyTorch Code Example |
---|---|---|
Supervised Learning | model = tf.keras.Sequential([tf.keras.layers.Dense(10, input_shape=(1,)), tf.keras.layers.Dense(1)]) | model = torch.nn.Sequential(torch.nn.Linear(1, 10), torch.nn.Linear(10, 1)) |
Unsupervised Learning | model = tf.keras.Sequential([tf.keras.layers.Dense(10, input_shape=(1,)), tf.keras.layers.Dense(1)]) | model = torch.nn.Sequential(torch.nn.Linear(1, 10), torch.nn.Linear(10, 1)) |
Reinforcement Learning | agent = tf.keras.optimizers.Adam(lr=0.001) | agent = torch.optim.Adam(lr=0.001) |
As seen above, TensorFlow and PyTorch provide similar functionality for implementing AI development techniques. The choice of framework largely depends on the specific requirements of the project and the developer’s level of expertise.
Overall, understanding the various AI development techniques is crucial for creating effective and efficient AI applications. Developers should strive to stay up-to-date with the latest developments in this rapidly evolving field to ensure that their AI applications remain competitive and relevant.
Implementing AI Coding Best Practices
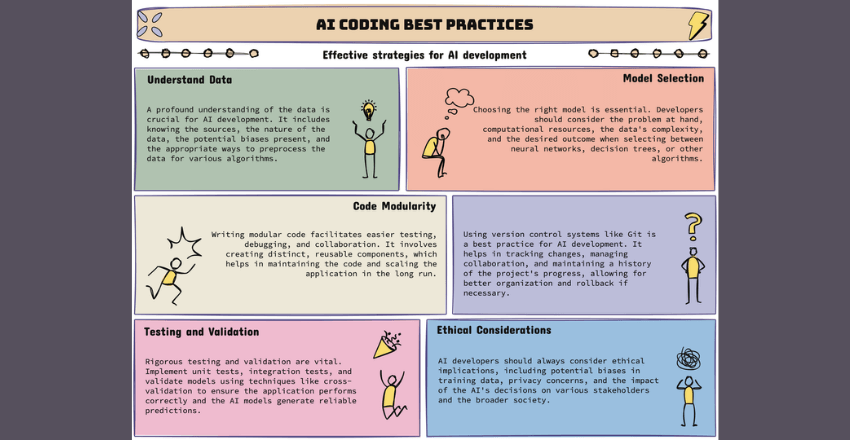
When developing AI applications, following coding best practices is crucial for ensuring efficient and accurate results.
Modular Programming
Modular programming involves breaking down a program into smaller, reusable modules that can be easily maintained and updated. In AI development, this practice can improve code organization and make it easier to scale or modify a project. By encapsulating code functionality into separate modules, maintaining complex AI projects can become less daunting.
Code Documentation
Proper documentation ensures that code can be clearly understood and maintained by developers over time. In AI development, this is especially important because of the complexity of the algorithms involved. Writing clear and concise comments and documentation enables developers to understand the inner workings of your code and make changes as needed, increasing project longevity.
Version Control
Version control is the practice of tracking and managing changes made to code over time. This practice is critical in AI development to allow for collaboration and to ensure that changes made to code do not break the project. Popular version control systems include Git and SVN.
Testing
Testing is an essential part of software development, and AI development is no exception. Proper testing ensures reliable results and can prevent costly errors in production. Effective testing involves creating a suite of test cases that check all aspects of the code, including edge cases and error handling.
By following these coding best practices in AI development, developers can improve the quality and efficiency of their code, leading to better results and reduced project time.
Comparing AI Frameworks: Code Examples in TensorFlow and PyTorch
When it comes to AI development, choosing the right framework is critical to the success of your project. Two of the most popular frameworks are TensorFlow and PyTorch, each with their own strengths and weaknesses.
TensorFlow is a widely-used open-source framework that has a robust ecosystem of tools and resources. It offers a vast array of pre-built functions and is known for its scalability and ability to handle large datasets. On the other hand, PyTorch is known for its user-friendliness and flexibility. It is often favored by researchers due to its ease of use in prototyping and ability to build complex neural networks.
Let’s take a look at how the same AI task can be implemented differently in these two frameworks.
Task: Creating a Simple Neural Network
We’ll create a simple neural network that has one input layer, one hidden layer, and one output layer. This network will be designed to perform a basic classification task.
TensorFlow Code Example
import tensorflow as tf
# Define model parameters
n_input = 784 # Number of input nodes (e.g., for MNIST dataset)
n_hidden = 64 # Number of hidden nodes
n_output = 10 # Number of output nodes (e.g., for MNIST dataset classes)
# Build the model
model = tf.keras.Sequential([
tf.keras.layers.Dense(n_hidden, input_shape=(n_input,), activation='relu'),
tf.keras.layers.Dense(n_output, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Summary of the model
model.summary()
In TensorFlow, the model is built using the Sequential
API, which is a linear stack of layers. The model is then compiled with an optimizer, a loss function, and a list of metrics.
PyTorch Code Example
import torch
import torch.nn as nn
import torch.nn.functional as F
# Define the network structure
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(784, 64) # Input to hidden layer
self.fc2 = nn.Linear(64, 10) # Hidden to output layer
def forward(self, x):
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
# Initialize the model
model = SimpleNN()
# Define loss function and optimizer
loss_function = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters())
print(model)
PyTorch uses a more flexible approach where you define a model class that inherits from nn.Module
. You specify the layers in the __init__
method and how data will pass through the network in the forward
method. The loss function and optimizer are defined outside the model class.
Comparison
- Flexibility: PyTorch offers more flexibility in designing complex models because of its dynamic computation graph. TensorFlow’s graph is static, but this has improved with the introduction of Eager Execution.
- Ease of Use: PyTorch is often praised for its simplicity and Pythonic way of implementation, making it a go-to for beginners and researchers. TensorFlow, with its comprehensive ecosystem and support, is highly scalable and preferred for production environments.
- Community and Support: TensorFlow, being older, has a larger community and more resources. PyTorch has gained rapid popularity, especially in the academic and research community, for its ease of use and simplicity.
Both frameworks have made significant strides in usability and performance. The choice between TensorFlow and PyTorch often comes down to personal preference, project requirements, and the specific features you need for your AI development tasks.
Optimizing AI Development with Efficient Project Management
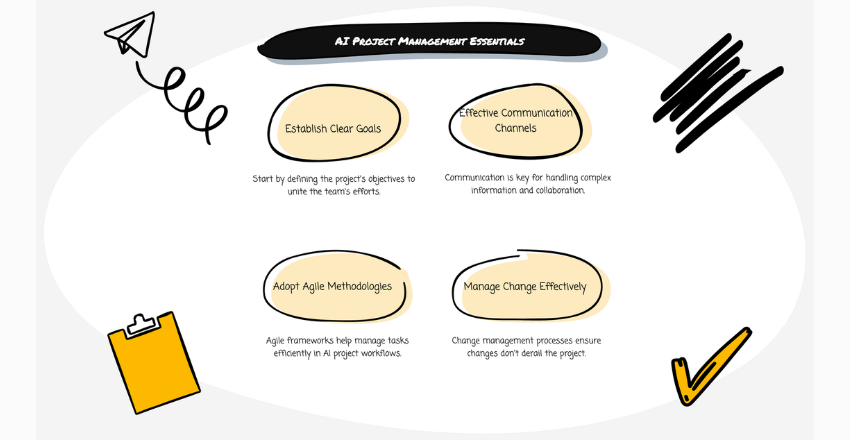
AI development projects require a disciplined approach to project management in order to ensure successful outcomes. Below we provide some best practices for effective AI project management.
Establish Clear Goals
Define clear goals and objectives at the outset of an AI development project. To avoid confusion and wasted time, make sure that everyone involved understands the goals and deliverables, and what is expected of them.
Effective Communication Channels
Communication is critical in any project management process, and AI development is no exception. Establish efficient communication channels to ensure that everyone involved has access to the information they need, and that any issues or concerns can be addressed in a timely manner.
Adopt Agile Methodologies
Agile methodologies enable teams to manage complex development projects more effectively by breaking the project down into smaller, manageable tasks. Adopting agile methodologies will ensure that your team can stay on track by monitoring progress and adjusting course as necessary.
Manage Change Effectively
Change is inevitable in any AI development project. To ensure that changes are managed effectively, establish a change management process to assess the impact of any changes, and identify strategies to mitigate any negative impacts.
Measure Progress
It’s essential to measure progress in order to ensure that your AI development project stays on track. Establish clear metrics for measuring progress and use them to track progress and identify any issues or areas that need attention.
By following these best practices for AI project management, you can optimize the development process and ensure successful outcomes for your AI projects.
Maintaining AI Code Quality: Best Practices for Software Development
When it comes to AI development, maintaining code quality is crucial for ensuring accuracy, efficiency, and scalability. In this section, we will cover some best practices for maintaining AI code quality, including coding standards, code review processes, and documentation practices.
Coding Standards
Following coding standards is important for ensuring consistency and readability in AI code. These standards can include guidelines for naming conventions, indentation, and commenting. Adhering to coding standards can also make it easier for other developers to understand and work with the code.
Some popular coding standards for AI development include Google’s TensorFlow style guide and the PyTorch code style guide.
Code Review Processes
Implementing a code review process can help catch errors and ensure that code adheres to coding standards. Code reviews can involve multiple reviewers and can be performed either automatically or manually. Automated reviews can be done using tools like linters and style checkers, while manual reviews involve a human reviewer analyzing the code for errors and adherence to best practices.
Code reviews can also provide opportunities for knowledge sharing and collaboration among team members.
Documentation Practices
Documenting AI code is important for enabling others to understand and work with the code. This documentation can include comments within the code itself, as well as external documentation such as user guides and API documentation.
Good documentation practices can also help with debugging and troubleshooting issues that arise in the code. Some popular documentation tools for AI development include Sphinx, Doxygen, and Javadoc.
By following these best practices for maintaining AI code quality, developers can ensure that their code is accurate, efficient, and scalable.
Enhancing AI Performance: Tips for Optimal AI Development

Artificial Intelligence (AI) development is a complex and constantly evolving field that requires careful attention to detail. To ensure optimal outcomes, developers need to adopt best practices and coding tips that enhance AI performance. Here are some techniques you can use:
- Optimize your AI model: Ensure that your AI model is optimized by selecting relevant features and making use of dimensionality reduction techniques to minimize the number of input nodes.
- Use hyperparameter tuning: Hyperparameters control the learning rate and regularization of your neural network. Adjusting these can enhance the accuracy of your AI model.
- Employ feature engineering: Feature engineering is the process of transforming raw data to extract relevant features that enhance the performance of your AI model. This can involve feature scaling, normalization, and extraction.
- Monitor your AI model: Continuously monitor your AI model’s performance by using learning curves and confusion matrices. This helps identify potential issues and fine-tune your AI model.
- Make use of transfer learning: Transfer learning involves using a pre-trained model to solve a related problem. This can significantly reduce the computational effort required to train your AI model.
- Use the right hardware: The choice of hardware can play a significant role in AI performance. GPUs are typically faster than CPUs when it comes to AI development.
Code Example: Hyperparameter Tuning in TensorFlow
Here’s an example of how hyperparameter tuning can enhance the accuracy of your AI model:
import tensorflow as tf
from tensorflow import keras
# Load data
data = keras.datasets.mnist.load_data()
train_images = data[0][0]
train_labels = data[0][1]
# Define model
model = keras.Sequential([
keras.layers.Flatten(input_shape=(28,28)),
keras.layers.Dense(128, activation='relu'),
keras.layers.Dense(10)
])
# Define hyperparameters
learning_rate = 0.01
batch_size = 128
epochs = 10
# Define optimizer
optimizer = keras.optimizers.Adam(learning_rate=learning_rate)
# Compile model
model.compile(optimizer=optimizer,
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
# Train model
model.fit(train_images, train_labels, epochs=epochs, batch_size=batch_size, verbose=2)
In this example, we adjust the hyperparameters of the neural network model by changing the learning rate, batch size, and number of epochs. We use the Adam optimizer, which adapts the learning rate based on the gradients of the loss function.
By experimenting with different hyperparameters, you can fine-tune your AI model and achieve better accuracy.
Final Thoughts
Adopting best practices in AI development is crucial for optimal project outcomes. AI coding best practices, best AI project practices, and adhering to AI software development guidelines are critical to achieve the desired results in AI development.
External Resources
https://www.ibm.com/topics/natural-language-processing
FAQ
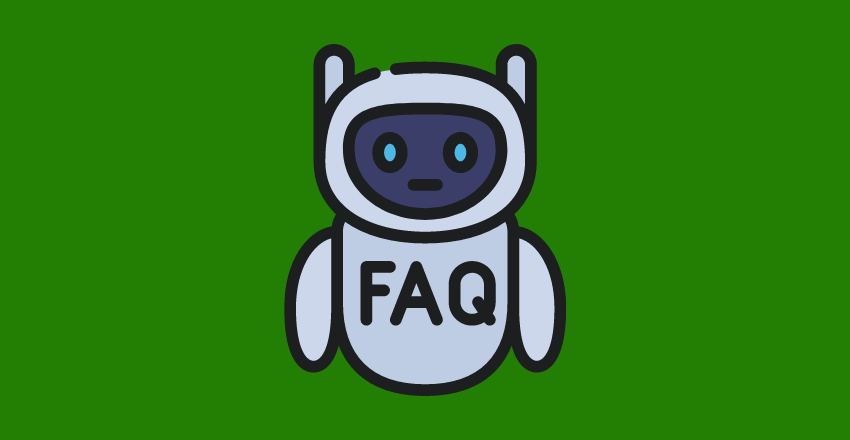
FAQ 1: How do I ensure the quality of data for AI model training?
Answer:
Quality data is crucial for AI success. Begin with data cleaning. Remove duplicates, handle missing values, and normalize data. Here’s a Python example using Pandas:
import pandas as pd
# Load your dataset
data = pd.read_csv('dataset.csv')
# Clean your data
data.drop_duplicates(inplace=True)
data.fillna(0, inplace=True) # Handling missing values by replacing them with 0
# Normalize data (Example: Min-Max normalization)
data = (data - data.min()) / (data.max() - data.min())
This ensures your AI model trains on clean, reliable data. Always assess and prepare your data before training for better accuracy and performance.
FAQ 2: What’s a good practice for splitting datasets in AI development?
Answer:
Splitting your dataset correctly is vital. Use a training set for model training, a validation set for tuning, and a test set for final evaluation. Here’s how to do it with Scikit-learn:
from sklearn.model_selection import train_test_split
# Assuming X is your features and y is your labels
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
X_train, X_val, y_train, y_val = train_test_split(X_train, y_train, test_size=0.25, random_state=42) # 0.25 x 0.8 = 0.2
This approach ensures your model can generalize well to new data, not just memorize the training data.
FAQ 3: How do I address overfitting in AI models?
Answer:
Overfitting is when a model learns the training data too well, performing poorly on unseen data. Regularization and dropout are effective strategies. Here’s a TensorFlow example:
import tensorflow as tf
from tensorflow.keras.layers import Dense, Dropout
from tensorflow.keras.models import Sequential
model = Sequential([
Dense(64, activation='relu', input_shape=(X_train.shape[1],)),
Dropout(0.5), # Dropout layer to prevent overfitting
Dense(1, activation='sigmoid')
])
model.compile(optimizer=’adam’, loss=’binary_crossentropy’, metrics=[‘accuracy’])
Dropout randomly sets input units to 0 at each step during training, helping prevent overfitting. Regularly evaluate your model’s performance on the validation set to monitor for overfitting.
Adopting these best practices in AI development ensures your models are accurate, robust, and reliable.

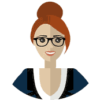
Jane Watson is a seasoned expert in AI development and a prominent author for the “Hire AI Developer” blog. With over a decade of experience in the field, Jane has established herself as a leading authority in AI app and website development, as well as AI backend integrations. Her expertise extends to managing dedicated development teams, including AI developers, Machine Learning (ML) specialists, and other supporting roles such as QA and product managers. Jane’s primary focus is on providing professional and experienced English-speaking AI developers to companies in the USA, Canada, and the UK.
Jane’s journey with AI began during her time at Duke University, where she pursued her studies in computer science. Her passion for AI grew exponentially as she delved into the intricacies of the subject. Over the years, she honed her skills and gained invaluable experience working with renowned companies such as Activision and the NSA. These experiences allowed her to master the art of integrating existing systems with AI APIs, solidifying her reputation as a versatile and resourceful AI professional.
Currently residing in the vibrant city of Los Angeles, Jane finds solace in her role as an author and developer. Outside of her professional pursuits, she cherishes the time spent with her two daughters, exploring the beautiful hills surrounding the city. Jane’s dedication to the advancement of AI technology, combined with her wealth of knowledge and experience, makes her an invaluable asset to the “Hire AI Developer” team and a trusted resource for readers seeking insights into the world of AI.