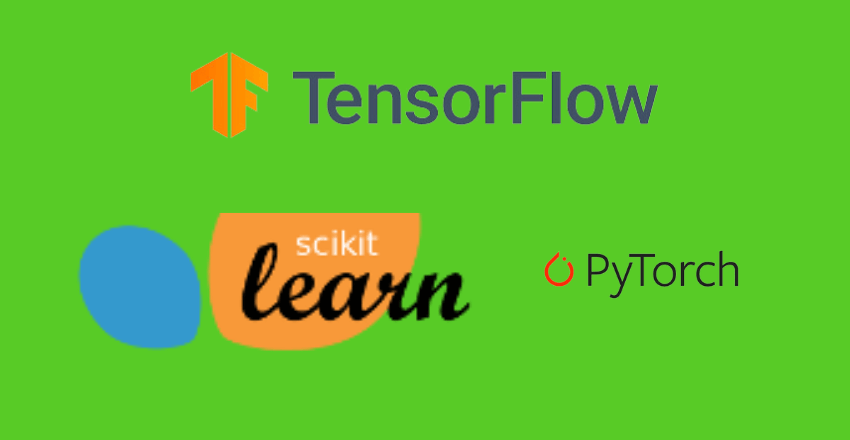
Best AI Frameworks for Python empower developers, simplifying complex tasks. Dive into TensorFlow, PyTorch, and Scikit-learn for groundbreaking innovations.
Believing that TensorFlow is the only viable AI framework for Python is a gross misconception.
Despite its popularity and wide usage, TensorFlow can often prove to be too complex and overwhelming for beginners.
AI Frameworks
AI development in Python is growing at an exponential rate, and selecting the right Python AI development frameworks is crucial for programmers. AI frameworks in Python provide a platform for creating and deploying AI models, and they can greatly enhance the efficiency and accuracy of your programming efforts.
Python AI development frameworks offer a wide range of benefits, including ease of use, flexibility, and the ability to work with large data sets. These frameworks are essential for developers who want to create innovative AI models that can be used in multiple applications, from machine learning to deep learning.
Python AI development frameworks can be used to create cutting-edge models that have the ability to recognize images, understand natural language, and even predict user behavior. Some of the top Python AI development frameworks include TensorFlow, PyTorch, Keras, Scikit-learn, Theano, and MXNet.
TensorFlow
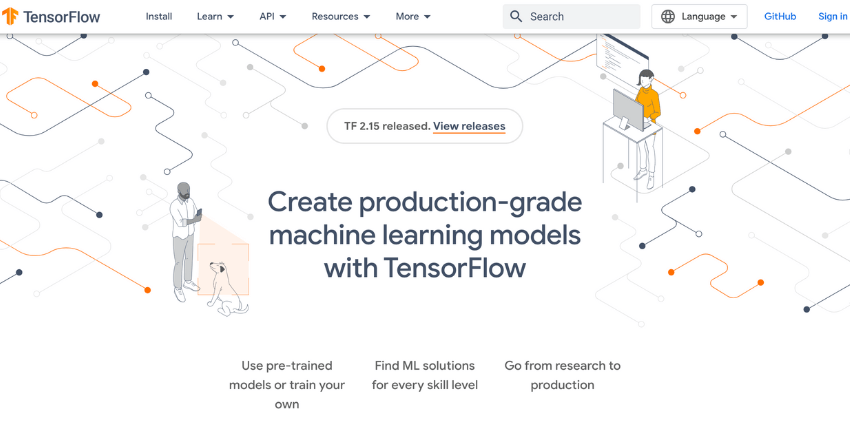
TensorFlow is one of the most popular Python AI frameworks, known for its flexibility and efficient data processing capabilities. It offers a vast range of tools and features that enable developers to build and train complex machine learning models.
TensorFlow provides APIs for low-level programming, allowing developers to control every aspect of the model-building process. It also offers high-level APIs, such as Keras, which simplifies the process of building and training neural networks.
One of the key strengths of TensorFlow is its ability to implement distributed computing, allowing users to train their models across multiple devices. The framework is also capable of deploying models across a variety of platforms, including desktops, mobile devices, and the cloud.

How do I start with a simple neural network example?
Answer: TensorFlow is an open-source AI framework developed by Google. It’s known for its flexibility and vast support for deep learning algorithms. TensorFlow allows for the creation of complex neural networks with an intuitive API.
Code Sample:
import tensorflow as tf
# Define a simple sequential model
model = tf.keras.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)), # Input layer
tf.keras.layers.Dense(128, activation='relu'), # Hidden layer
tf.keras.layers.Dropout(0.2),
tf.keras.layers.Dense(10) # Output layer
])
# Compile the model
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
# Model summary
model.summary()
Explanation: This code snippet creates a basic neural network for classification with TensorFlow’s Keras API. It starts with a flattening layer to reshape input data, followed by a dense (fully connected) layer with ReLU activation, a dropout layer for regularization, and finally, a dense output layer.
The model is compiled with the Adam optimizer and a loss function suitable for classification tasks.
Overall, TensorFlow is an excellent choice for Python AI toolkit selection, providing a range of features that enable developers to build and train complex models for a wide variety of use cases.
PyTorch
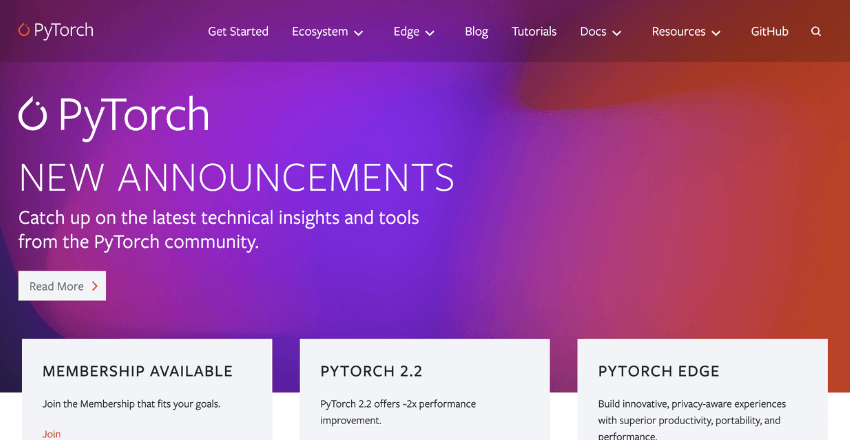
PyTorch is a Python framework for building and training neural networks. It is known for its simplicity and ease of use, making it a popular choice for many developers. It was developed by Facebook’s AI research group and has gained a lot of attention in recent years.
One of the standout features of PyTorch is its dynamic computation graph. This allows developers to modify the network architecture on the fly, making it easy to experiment with different configurations. It also makes debugging easier as errors can be tracked more easily.
PyTorch also boasts a strong community and support for a wide range of platforms, including GPUs and mobile devices. It also integrates well with other Python libraries such as NumPy, SciPy, and Pandas.
When comparing PyTorch to TensorFlow, one of the main differences is in the way the two frameworks handle computation graphs. PyTorch uses a dynamic computation graph, while TensorFlow uses a static computation graph. This means that PyTorch is better suited for research and experimentation, while TensorFlow is better suited for production environments.
Here is an example of how to create a simple neural network using PyTorch:
# Import necessary libraries
import torch
import torch.nn as nn
import torch.optim as optim
# Define network architecture
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(10, 5)
self.fc2 = nn.Linear(5, 1)
def forward(self, x):
x = torch.sigmoid(self.fc1(x))
x = torch.sigmoid(self.fc2(x))
return x
# Create network object
net = Net()
# Define loss function and optimizer
criterion = nn.MSELoss()
optimizer = optim.SGD(net.parameters(), lr=0.01)
# Train the network
for epoch in range(100):
optimizer.zero_grad()
output = net(input)
loss = criterion(output, target)
loss.backward()
optimizer.step()
Overall, PyTorch is an excellent choice for developers looking for a Python framework for AI development. Its dynamic computation graph, ease of use, and strong community make it a top contender in the Python AI ecosystem.
Keras
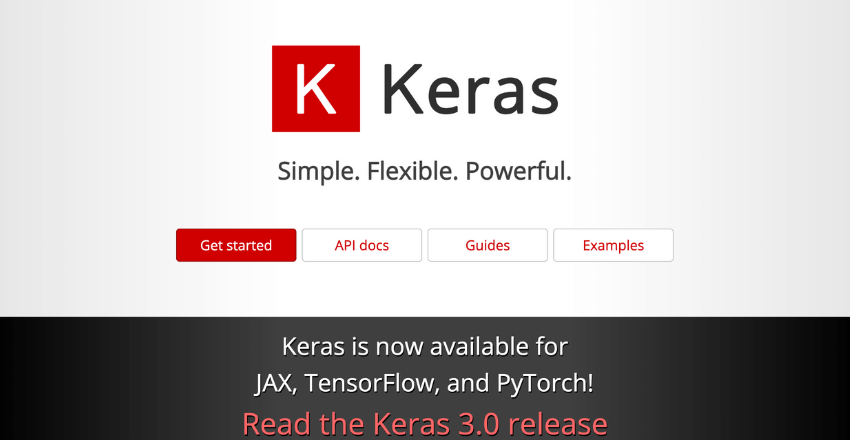
When it comes to Python AI development frameworks, Keras stands out as one of the most user-friendly tools available. Built on top of TensorFlow, Keras provides a simplified interface that makes it easy for developers to build complex neural networks.
With Keras, you can quickly prototype and develop deep learning models, thanks to its intuitive and modular architecture. Keras also offers a wide range of pre-built neural network layers and models, making it an excellent choice for both beginners and experienced developers.
One of the primary advantages of Keras is its speed and flexibility. Whether you’re working on a small scale or a large project, Keras can easily scale to meet your needs. Additionally, Keras supports both convolutional and recurrent neural networks, making it useful for a wide range of applications.
Keras also offers a range of advanced features, such as automatic differentiation, GPU support, and distributed training. These features help streamline the development process and make it easier to work with large datasets.
Overall, Keras is an excellent tool for Python AI development frameworks. Its simplicity, speed, and flexibility make it a top choice for developers of all skill levels.
Scikit-learn
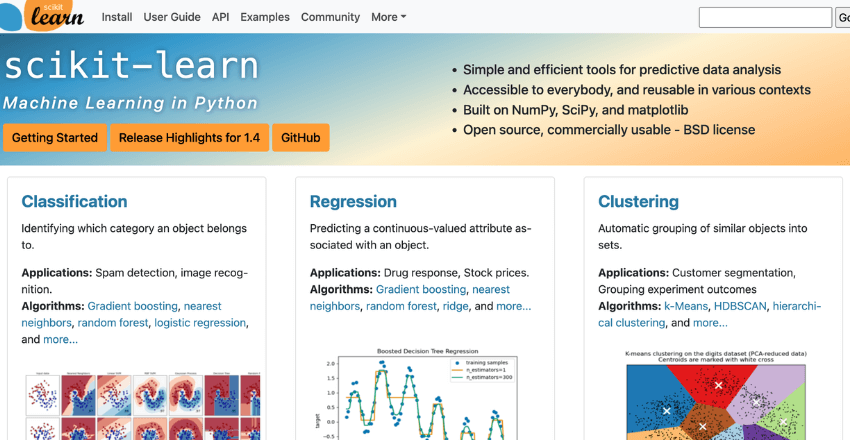
Scikit-learn is a powerful and user-friendly library for machine learning in Python. It is built on top of NumPy and SciPy, providing a wide range of algorithms for classification, regression, and clustering tasks. With easy-to-use interfaces and extensive documentation, Scikit-learn is an excellent choice for developers with varying levels of experience.
One of the key strengths of Scikit-learn is its ability to handle both supervised and unsupervised learning tasks. It supports a variety of techniques for data preprocessing, dimensionality reduction, and model selection. Additionally, it provides tools for model evaluation, allowing developers to fine-tune their models and optimize their performance.
Scikit-learn has a vibrant community and is used extensively in both academia and industry. Its popularity can be attributed to its simplicity and effectiveness, making it a top choice for developers building machine learning models.
- Example Code: Here is an example of Scikit-learn’s implementation of a decision tree classifier:
from sklearn.tree import DecisionTreeClassifier
clf = DecisionTreeClassifier()
clf.fit(X_train, y_train)
predictions = clf.predict(X_test)
Scikit-learn also provides a range of other algorithms, such as k-means clustering and support vector machines, with similarly easy-to-use syntax.
Industry Example: A study conducted by IBM found that Scikit-learn was the most popular machine learning library among data scientists, with almost 50% of respondents reporting it as their primary tool for model development (source).
Theano: A High-performance Numerical Computation Library for AI Development in Python
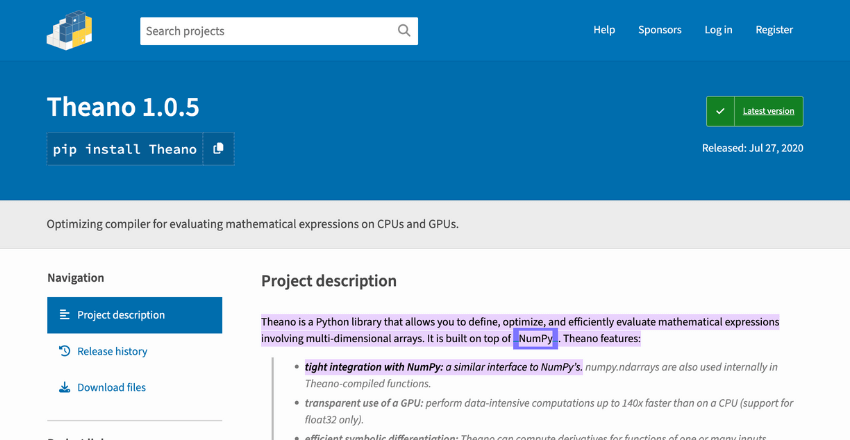
Python has become one of the most popular programming languages for artificial intelligence (AI) development, thanks to its simplicity and flexibility. Theano is a high-performance numerical computation library that has contributed significantly to the popularity of AI development in Python.
Theano is widely used for developing deep neural networks, and it offers an excellent platform for researchers and developers working on machine learning algorithms. It is also an open-source library and is available under the BSD license.
Theano can be used to perform various numerical computations efficiently, including matrix operations, symbolic differentiation, and optimization. It simplifies the process of creating complex mathematical expressions and allows users to focus on the development of algorithms.
Theano vs. Other AI Frameworks
Choosing the right AI framework can be challenging, especially for those new to AI development. When selecting a framework, developers must consider factors such as ease of use, performance, and community support.
Theano may not be as user-friendly as some other libraries, such as Keras, but it offers excellent performance and flexibility. Its symbolic differentiation abilities set it apart from other libraries and make it a popular choice among researchers developing complex algorithms.
Other popular AI frameworks for Python, such as TensorFlow and PyTorch, also offer advanced capabilities and are often compared to Theano. However, each framework has its unique strengths and weaknesses, making it important for developers to consider their specific needs before selecting a framework.
Code Example for Theano
import theano
import theano.tensor as T
import numpy as np
# Define the symbolic variables
x = T.matrix('x')
y = T.matrix('y')
# Define the expression
z = T.dot(x, y)
# Compile the function
matrix_multiply = theano.function(inputs=[x, y], outputs=z)
# Test with numpy arrays
x_val = np.array([[1, 2], [3, 4]], dtype=theano.config.floatX)
y_val = np.array([[5, 6], [7, 8]], dtype=theano.config.floatX)
# Execute the function
result = matrix_multiply(x_val, y_val)
print("Matrix multiplication result:\n", result)
This code snippet showcases Theano’s ability to define and optimize mathematical expressions. It uses symbolic programming to define a matrix multiplication operation, compiles it into a callable function, and then executes this function with NumPy arrays as inputs. This process demonstrates Theano’s power in handling complex mathematical computations efficiently, making it a valuable tool for AI research and development.
Comparing Theano with other AI frameworks:
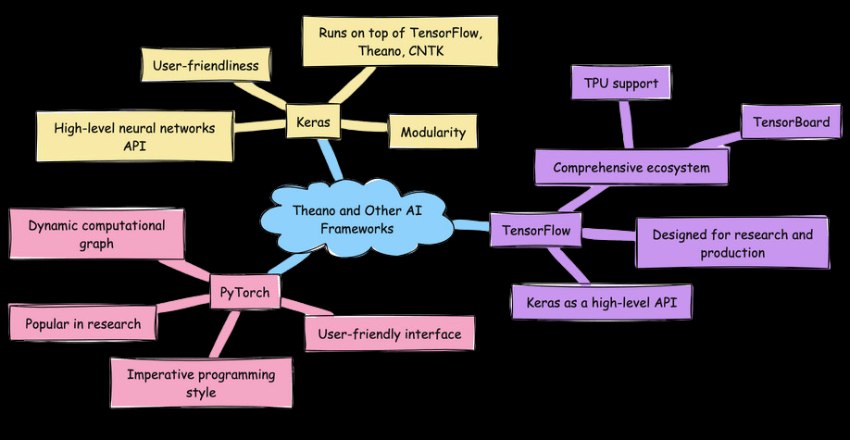
- TensorFlow: Offers a comprehensive ecosystem including TensorBoard for visualization, TPU support for accelerated computing, and Keras as a high-level API. TensorFlow is designed for both research and production.
- PyTorch: Known for its dynamic computational graph and user-friendly interface, making it particularly popular in research. PyTorch provides an imperative programming style that feels more like traditional Python coding.
- Keras: Acts as a high-level neural networks API running on top of TensorFlow, Theano, and CNTK. Keras is designed for human beings, not machines, emphasizing user-friendliness and modularity.
While Theano offers excellent performance and flexibility, especially with symbolic differentiation, it has been officially discontinued in terms of new developments since 2017. Developers often choose TensorFlow or PyTorch for more active community support and ongoing development, whereas Keras offers an easier entry point for beginners with the flexibility to switch between backends. Each framework has its niche, catering to different preferences and project requirements.
Theano offers a unique approach to defining and optimizing mathematical expressions in Python. Here is a simple example of how to use Theano to compute the value of a matrix multiplication:
Theano is a powerful library for numerical computation and has been instrumental in the success of AI development in Python. Its ability to efficiently perform complex mathematical operations makes it a popular choice among researchers and developers working on machine learning algorithms.
When selecting an AI framework for Python, developers must consider their specific needs, including performance, ease of use, and community support. Theano offers advanced capabilities and is often compared to other popular libraries, such as TensorFlow and PyTorch.
External Resources
https://scikit-learn.org/stable/
https://scikit-learn.org/stable/
Final Thoughts
Choosing the best AI frameworks for Python can make a significant impact on the development of your AI projects. In this article, we have highlighted some of the top-rated tools available in the Python AI ecosystem, providing code examples to illustrate their features and capabilities.
Overall, the best AI frameworks for Python depend on your specific requirements and preferences. We hope this article has provided valuable insights into selecting the best Python AI toolkit for your next project.
FAQ
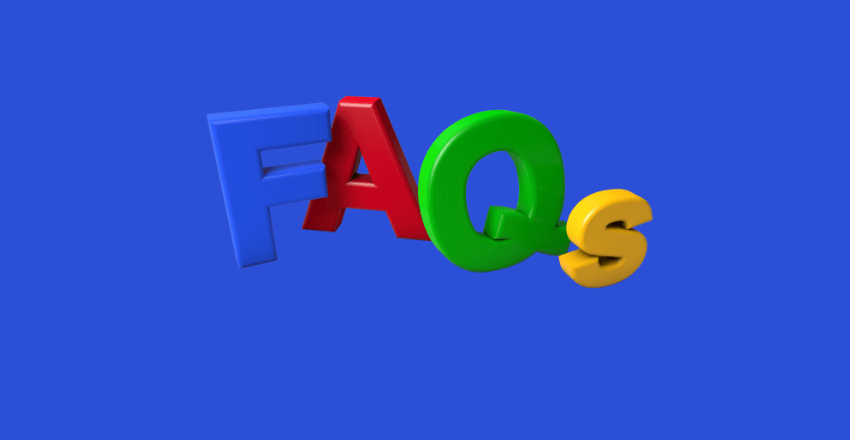
1. How does TensorFlow facilitate deep learning model development?
Answer: TensorFlow shines in its comprehensive ecosystem. It supports a wide array of tools for model building, training, and deployment, making it a go-to for professionals. Its dynamic computational graph allows for intuitive model adjustments.
Code Sample:
import tensorflow as tf
# Define a simple model
model = tf.keras.Sequential([
tf.keras.layers.Dense(10, activation='relu', input_shape=(784,)),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# Summary of the model
model.summary()
Explanation: This snippet outlines creating a basic neural network using TensorFlow’s Keras API. It’s designed for a 784-dimensional input, featuring two layers with ReLU and softmax activations. TensorFlow simplifies model compilation and evaluation, showcasing its user-friendly approach.
2. What makes PyTorch a favorite for dynamic model experimentation?
Answer: PyTorch offers an imperative programming model, making it intuitive for Python users. Its dynamic computation graph enables modifications on-the-fly, a boon for research and development phases.
Code Sample:
import torch
import torch.nn as nn
# Define a simple neural network
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.layer1 = nn.Linear(784, 128)
self.layer2 = nn.Linear(128, 10)
def forward(self, x):
x = torch.relu(self.layer1(x))
return torch.softmax(self.layer2(x), dim=1)
# Instantiate the model
model = SimpleNN()
print(model)
Explanation: Here, a basic neural network is crafted with PyTorch, showcasing its straightforward syntax. The model inherits from nn.Module
, with a forward method defining data flow. This exemplifies PyTorch’s flexibility in model experimentation.
3. Why is Scikit-learn considered a great starting point for AI newcomers?
Answer: Scikit-learn excels in simplicity and accessibility. It provides a wide range of tools for machine learning that are easy to use, making it ideal for beginners. Its comprehensive documentation aids in learning.
Code Sample:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# Load the iris dataset
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2)
# Train a Random Forest Classifier
clf = RandomForestClassifier(n_estimators=100)
clf.fit(X_train, y_train)
# Evaluate the model
accuracy = clf.score(X_test, y_test)
print(f"Model Accuracy: {accuracy}")
Explanation: This example demonstrates using Scikit-learn to train a Random Forest classifier on the iris dataset. It emphasizes Scikit-learn’s user-friendly API, from data loading to model training and evaluation, illustrating its suitability for beginners entering the AI field.
Each framework has its forte: TensorFlow’s extensive ecosystem, PyTorch’s dynamic experimentation, and Scikit-learn’s simplicity. Choosing the right framework depends on your project requirements, experience level, and the specific AI challenges you aim to tackle.

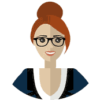
Jane Watson is a seasoned expert in AI development and a prominent author for the “Hire AI Developer” blog. With over a decade of experience in the field, Jane has established herself as a leading authority in AI app and website development, as well as AI backend integrations. Her expertise extends to managing dedicated development teams, including AI developers, Machine Learning (ML) specialists, and other supporting roles such as QA and product managers. Jane’s primary focus is on providing professional and experienced English-speaking AI developers to companies in the USA, Canada, and the UK.
Jane’s journey with AI began during her time at Duke University, where she pursued her studies in computer science. Her passion for AI grew exponentially as she delved into the intricacies of the subject. Over the years, she honed her skills and gained invaluable experience working with renowned companies such as Activision and the NSA. These experiences allowed her to master the art of integrating existing systems with AI APIs, solidifying her reputation as a versatile and resourceful AI professional.
Currently residing in the vibrant city of Los Angeles, Jane finds solace in her role as an author and developer. Outside of her professional pursuits, she cherishes the time spent with her two daughters, exploring the beautiful hills surrounding the city. Jane’s dedication to the advancement of AI technology, combined with her wealth of knowledge and experience, makes her an invaluable asset to the “Hire AI Developer” team and a trusted resource for readers seeking insights into the world of AI.