How to Add AI to Your App can set you apart from competitors. Simple steps to integrate AI for smarter functionality.
Integrating artificial intelligence (AI) into mobile applications has become increasingly popular in recent years. As the demand for smart and personalized apps continues to grow, developers are turning to AI to enhance their products. Adding AI to your app can improve user experience, increase efficiency, and provide valuable insights.
AI in App Development
Artificial Intelligence (AI) is a branch of computer science that involves the creation of intelligent machines that can learn and perform tasks that typically require human intelligence, such as recognizing speech, understanding natural language, and making decisions.
Integrating AI in mobile apps has become increasingly popular in recent years due to its potential to enhance user experiences and increase engagement.
Adding AI to applications involves incorporating various AI technologies, such as Natural Language Processing (NLP), Machine Learning (ML), and Computer Vision (CV), into mobile applications. These technologies enable apps to understand and analyze user behavior, personalize content and recommendations, and automate routine tasks.
By incorporating AI into mobile applications, businesses can gain insights into user behavior and preferences, deliver personalized content and recommendations, and streamline operations. AI can also help businesses save costs by automating routine tasks and reducing the need for manual labor.
Types of AI Applications
There are several types of AI applications that can be integrated into mobile apps:
- Natural Language Processing (NLP): This involves the ability of machines to understand and interpret human language. NLP is commonly used in chatbots and virtual assistants, enabling them to respond to user queries and perform tasks.
- Machine Learning (ML): This involves the ability of machines to learn from data without being explicitly programmed. ML is commonly used in predictive analytics and recommendation systems, enabling apps to provide personalized content and recommendations to users.
- Computer Vision (CV): This involves the ability of machines to interpret and analyze images and videos. CV is commonly used in object detection and recognition, enabling apps to identify and classify objects in the real world.
By understanding the different types of AI applications, businesses can choose the right AI technologies to integrate into their mobile apps.
Choosing the Right AI Framework or Language
Choosing the right AI framework or language for your app is crucial to ensure effective AI app implementation. There are various frameworks and languages to choose from depending on your requirements, so it’s essential to understand the nuances of each.
Python
Python is one of the most widely used languages for AI app development due to its simplicity and ease of use. It offers several AI libraries, including TensorFlow, Keras, and PyTorch, and provides powerful data analysis capabilities.
Here’s a code example demonstrating how to train a simple neural network using TensorFlow:
import tensorflow as tf
from tensorflow import keras
model = keras.Sequential([keras.layers.Dense(units=1, input_shape=[1])])
model.compile(optimizer='sgd', loss='mean_squared_error')
xs = [-1, 0, 1, 2, 3, 4]
ys = [-3, -1, 1, 3, 5, 7]
model.fit(xs, ys, epochs=500)
print(model.predict([10.0]))
Java
Java has several AI libraries such as Deeplearning4j and TensorFlow Java and is commonly used for building enterprise-level AI applications. Java offers advanced memory management and security features, making it the language of choice for developing large-scale apps. Here’s a code example demonstrating how to implement a simple AI chatbot using the Dialogflow API:
//set up the Dialogflow API credentials
String projectId = "PROJECT_ID";
String languageCode = "en-US";
SessionsSettings settings = SessionsSettings.newBuilder()
.setCredentialsProvider(FixedCredentialsProvider.create(credentials))
.build();
//set up the session and query input
String sessionId = UUID.randomUUID().toString();
SessionName session = SessionName.of(projectId, sessionId);
QueryInput queryInput = QueryInput.newBuilder().setText(TextInput.newBuilder().setText(inputString).setLanguageCode(languageCode)).build();
//detect intent and retrieve the Dialogflow response
try (SessionsClient sessionsClient = SessionsClient.create(settings)) {
DetectIntentResponse response = sessionsClient.detectIntent(session, queryInput);
QueryResult queryResult = response.getQueryResult();
String fulfillmentText = queryResult.getFulfillmentText();
}
Other popular AI languages and frameworks include R, MATLAB, and Caffe. It’s important to choose a language or framework that aligns with your requirements, development skills, and resources.
Preparing Your App for AI Integration
Integrating AI into your app requires careful preparation to ensure a smooth implementation. The following steps should be taken to effectively incorporate AI into your mobile application:
Collecting and Cleaning Data
The first step in preparing your app for AI integration is to gather and clean the necessary data. This data should be representative of the user behavior and preferences you plan to predict with your AI model. Once you have collected your data, the next step is to clean it, ensuring that the data is consistent and free from errors.
Example: In Python, you can use the Pandas library to clean and preprocess your data. Here is a code example that removes all non-numeric characters from a data column:
import pandas as pd dataframe = pd.read_csv("data.csv")
dataframe['column_name'] =
dataframe['column_name'].str.replace('\D+', '')
Preprocessing Data
Preprocessing your data involves applying transformations to make the data suitable for AI analysis. This can involve standardizing data, normalizing data, or transforming categorical data into numerical values. Preprocessing can also involve reducing the dimensionality of your data to focus on relevant features.
Example: In Python, you can use the Scikit-learn library to preprocess your data. Here is a code example that standardizes a dataset:
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler().fit(X_train)
X_train = scaler.transform(X_train)
Selecting the Right AI Tools
Choosing the right tools to implement AI features in your app is critical. There are a variety of AI frameworks and languages available, each with their own strengths and weaknesses. Popular frameworks include TensorFlow, PyTorch, and Keras.
Example: Comparing two frameworks, TensorFlow and PyTorch:
TensorFlow:
import tensorflow as tf
model = tf.keras.models.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dropout(0.2),
tf.keras.layers.Dense(10)
])
predictions = model(images)
PyTorch:
import torch import torch.nn as nn class NeuralNet(nn.Module):
def __init__(self): super(NeuralNet,
self).__init__() self.flatten = nn.Flatten() self.linear_relu_stack =
nn.Sequential( nn.Linear(784, 512), nn.ReLU(), nn.Dropout(0.2), nn.Linear(512, 10) )
def forward(self, x): x = self.flatten(x) logits = self.linear_relu_stack(x)
return logits model = NeuralNet()
By following these steps, you will be well on your way to effectively integrating AI into your mobile application, enhancing its capabilities and providing valuable predictive functionalities for your users.
Training Your AI Model
Before implementing AI features in your app, you need to train an AI model. This involves selecting the right training data, choosing an appropriate algorithm, and optimizing your model for performance.
Selecting the Right Training Data
The first step in training your AI model is selecting the right training data. The quality and quantity of your training data will have a significant impact on the accuracy of your model. You should ensure that your training data is diverse and representative of the real-world scenarios that your app will encounter.
For example, if you are developing a language translation app, you should use training data that includes a variety of languages, dialects, and writing styles. You can use publicly available datasets or create your own by scraping websites or collecting user-generated content.
Choosing an Appropriate Algorithm
Once you have collected your training data, you need to choose an appropriate algorithm for your AI model. There are several types of algorithms you could use, such as decision trees, linear regression, and neural networks.
The choice of algorithm will depend on the complexity of the problem you are trying to solve and the nature of your data. For example, if you are classifying images, a convolutional neural network (CNN) is a commonly used algorithm that is well-suited for image recognition tasks.
It’s important to note that you may need to try several algorithms before finding the one that works best for your app. You can use popular AI frameworks like TensorFlow, Keras, and PyTorch to build and train your model.
Optimizing Your AI Model
Once you have chosen your algorithm and trained your model, you need to optimize it for performance. This involves tweaking the parameters of your model to improve its accuracy and reduce its error rate.
One way to optimize your model is to use a technique called regularization, which adds a penalty term to the loss function that encourages simpler models over more complex ones. You can also use techniques like dropout, which randomly removes neurons from the model during training to prevent overfitting.
It’s important to test your model thoroughly before integrating it into your app. You can use test datasets to evaluate the performance of your model and adjust its parameters as necessary.
Implementing AI Features in Your App
Integrating AI features in your app requires careful consideration of your app’s architecture and functionality. Here are the key steps involved in adding AI to your app:
1. Integrating the Trained Model into Your App
After training your AI model, the next step is to integrate it into your app. This requires adding the model’s code into your app’s codebase. You should also consider the best approach to handling user inputs to ensure that the AI model can generate accurate outputs.
Example: In Python, you can use the TensorFlow library to integrate your trained AI model into your app’s codebase:
Code Sample (Python) |
---|
import tensorflow as tf model = tf.keras.models.load_model(‘model.h5’) |
2. Handling User Inputs
The accuracy of your AI model depends on the quality of the data it processes. Therefore, it’s important to handle user inputs to ensure that the data fed into the model is as clean and relevant as possible. This can be achieved through data validation and preprocessing techniques.
Example: In JavaScript, you can use regular expressions to validate user inputs:
Code Sample (JavaScript) |
---|
function validateInput(input) { const regex = /^[a-zA-Z]+$/; // Only allow letters return regex.test(input); } |
3. Displaying AI-Generated Outputs
Once your AI model has generated outputs, you need to display them in a user-friendly manner. This can be achieved through various methods, such as presenting them in a table or graph, or through natural language generation (NLG).
Example: In Python, you can use the Matplotlib library to generate a bar chart of AI-generated outputs:
Code Sample (Python) |
---|
import matplotlib.pyplot as plt labels = [‘Output 1’, ‘Output 2’, ‘Output 3’] values = [10, 20, 30] plt.bar(labels, values) plt.show() |
By following these steps, you can successfully add AI features to your app and enhance its functionality and user experience.
Testing and Evaluating Your AI-Enabled App
Testing and evaluating your AI-enabled app is a critical step in ensuring its success, both for the sake of your end-users and your business objectives. Here are some key considerations and steps to follow as you go through the testing and evaluation process:
Create Test Cases
One of the first things you’ll need to do is create test cases that reflect your app’s intended functionality, as well as a range of possible user scenarios and inputs. These test cases should include both “happy path” tests, which assume everything goes according to plan, as well as “edge case” tests that cover unexpected or unusual situations.
For example, if you’re building a chatbot app, you might create test cases that include common user queries, such as “What’s the weather like today?” or “How do I book a flight?” as well as less common requests, such as “What’s the meaning of life?” or “Tell me a joke.”
Measure Performance Metrics
Once you’ve created your test cases, you’ll need to measure your app’s performance against them to ensure it’s meeting your desired outcomes. Some key metrics to consider include:
- Accuracy: How often does your app correctly interpret and respond to user inputs?
- Speed: How quickly does your app respond to user inputs?
- Robustness: How well does your app handle unexpected or erroneous inputs?
It’s important to establish a baseline for these metrics before you begin testing, so you can accurately assess any improvements or issues that arise as you go through the testing process.
Optimize Your App Based on User Feedback
Finally, it’s crucial to consider user feedback as you refine and optimize your AI-enabled app. Providing users with a way to report issues or suggest improvements can help you catch issues early and ensure your app is meeting their needs. Be sure to take this feedback into account as you iterate on your app, making changes and improvements as needed.
By following these steps and taking testing and evaluation seriously, you can ensure your AI-enabled app is delivering the functionality and user experience you intended.
Addressing Ethical Considerations
Integrating AI into mobile applications offers a plethora of benefits, ranging from enhancing user experience to increasing app functionality. Nonetheless, AI development and deployment require a comprehensive understanding of ethical considerations that may arise during the process. In this section, we will explore some of the ethical considerations that must be addressed during AI app development.
Data Privacy
Data privacy represents one of the ethical considerations that must be addressed during AI app development. As the amount of data used for training AI models keeps increasing, it is essential to ensure that user data is collected, stored, and processed ethically. Developers must be transparent about data usage and obtain consent from users before collecting and processing their data.
“One of the key elements of AI ethical considerations is privacy, which can be addressed through the transparent and ethical collection and treatment of data.”
Algorithm Bias
Algorithm bias occurs when an AI-driven app discriminates against a particular group of users or produces unfavorable outcomes for an individual or group. Developers must ensure that their AI models are not biased and are trained using diverse sets of data. They must also monitor their AI models for any biases and correct them when necessary.
“AI technology has the potential to perpetuate social biases, which can be addressed by developing AI models with diverse datasets and eliminating any biases during the testing phase.”
Transparency in AI Decision-Making
Another ethical consideration that must be addressed during AI app development is transparency in AI decision-making. Developers must ensure that their AI models are transparent and that their decisions can be explained to users. They must also provide users with the option to contest AI-generated decisions.
“AI models that generate decisions that are not transparent raise ethical concerns. To address this, developers must ensure that their AI models are transparent and provide users with the option to contest AI-generated decisions.”
Optimizing and Scaling Your AI-Enhanced App
Once you have successfully integrated AI into your app development process, it is essential to optimize and scale your app to ensure its efficiency and reliability. Here are some tips and techniques to help you optimize and scale your AI-enhanced app:
1. Reduce Inference Time
One crucial factor to consider when optimizing your AI-enabled app is reducing inference time. Inference time is the time taken for the AI model to process incoming data and produce an output. The longer the inference time, the slower your app will run.
To reduce inference time, you can use techniques such as model quantization, model pruning, and model compression. These techniques help reduce the size and complexity of the AI model, making it faster and more efficient.
2. Handle Increased User Load
As your AI-enhanced app gains popularity, you may experience an increase in user load. To handle this increase, you need to ensure that your app can handle the additional traffic without crashing or slowing down.
You can use techniques such as load balancing, caching, and serverless computing to handle increased user load. These techniques help distribute the workload across multiple servers or cloud-based services, ensuring that your app can handle the increased traffic.
3. Implement Cloud-based AI Services
One way to scale your AI-enhanced app is to implement cloud-based AI services. Cloud-based AI services provide on-demand access to AI capabilities without the need for extensive infrastructure or hardware resources.
You can use cloud-based AI services such as Amazon Web Services (AWS), Google Cloud Platform (GCP), or Microsoft Azure to add AI functionality to your app. These services offer pre-built AI models, tools, and APIs that you can integrate into your app, reducing development time and cost.
Optimizing and scaling your AI-enabled app is essential for its success. By reducing inference time, handling increased user load, and implementing cloud-based AI services, you can ensure that your app runs smoothly and efficiently.
Final Thoughts
Incorporating AI into mobile app development is becoming increasingly important in today’s tech-driven world. By adding AI to your app, you can enhance its functionalities and stay ahead of the competition. In this article, we covered the steps involved in adding AI to your app, from selecting the right framework to optimizing and scaling your app.
External Resources
https://hbr.org/2023/07/how-to-train-generative-ai-using-your-companys-data
FAQ
FAQ 1: How do I integrate a chatbot into my mobile app using AI?
Answer: Adding a chatbot to your app can significantly improve user engagement by providing instant support and interactive experiences. One straightforward way to do this is by utilizing the Dialogflow API from Google. Dialogflow allows you to create and manage chatbot interactions that can easily be integrated into your mobile app. Here’s a basic example in Python to call a Dialogflow agent:
from google.api_core.exceptions import InvalidArgument
from google.cloud import dialogflow
import os
os.environ["GOOGLE_APPLICATION_CREDENTIALS"] = 'path/to/your/google-credentials.json'
DIALOGFLOW_PROJECT_ID = 'your-project-id'
DIALOGFLOW_LANGUAGE_CODE = 'en'
SESSION_ID = 'me'
session_client = dialogflow.SessionsClient()
session = session_client.session_path(DIALOGFLOW_PROJECT_ID, SESSION_ID)
text_input = dialogflow.TextInput(text="hello", language_code=DIALOGFLOW_LANGUAGE_CODE)
query_input = dialogflow.QueryInput(text=text_input)
try:
response = session_client.detect_intent(session=session, query_input=query_input)
except InvalidArgument:
raise
print("Query text:", response.query_result.query_text)
print("Detected intent:", response.query_result.intent.display_name)
print("Detected intent confidence:", response.query_result.intent_detection_confidence)
print("Fulfillment text:", response.query_result.fulfillment_text)
This code snippet demonstrates how to send a text query (“hello”) to your Dialogflow agent and receive a response. Make sure to replace "path/to/your/google-credentials.json"
, "your-project-id"
, and other placeholders with your actual project details.
FAQ 2: How can I add image recognition features to my app?
Answer: Image recognition can be integrated into your app to offer features like photo tagging, object detection, and more. TensorFlow Lite is a great tool for this, allowing you to run machine learning models on mobile devices. Here’s how you can use a pre-trained TensorFlow Lite model in your app:
First, convert your TensorFlow model to the TensorFlow Lite format. Then, integrate it into your mobile app project. For demonstration, here’s a pseudo-code snippet showing how to load and use a TensorFlow Lite model in an Android app:
try {
// Load the TFLite model
AssetFileDescriptor fileDescriptor = context.getAssets().openFd("model.tflite");
FileInputStream inputStream = new FileInputStream(fileDescriptor.getFileDescriptor());
FileChannel fileChannel = inputStream.getChannel();
long startOffset = fileDescriptor.getStartOffset();
long declaredLength = fileDescriptor.getDeclaredLength();
MappedByteBuffer buffer = fileChannel.map(FileChannel.MapMode.READ_ONLY, startOffset, declaredLength);
// Initialize the TensorFlow Lite interpreter
Interpreter tflite = new Interpreter(buffer);
// Prepare your input data
float[][] input = new float[1][inputSize]; // Adjust the size accordingly
// Fill the input array with your data
// Run inference
float[][] output = new float[1][outputSize]; // Adjust the size accordingly
tflite.run(input, output);
// Use the model output as needed
} catch (IOException e) {
// Handle exceptions
}
This Java snippet for Android apps illustrates how to load a TensorFlow Lite model and run inference with it. The model’s input and output sizes depend on the specific model you’re using.
FAQ 3: How can I use AI to personalize content in my app?
Answer: Personalizing content with AI can significantly enhance user satisfaction by delivering more relevant content to each user. One way to implement this is by using machine learning to analyze user interactions and preferences within your app. Here’s a Python example using the scikit-learn library to cluster users based on their preferences:
from sklearn.cluster import KMeans
import numpy as np
# Example data: user preferences on a scale from 1 to 5
user_preferences = np.array([
[5, 2, 3],
[1, 5, 4],
[2, 3, 5],
[4, 4, 1]
])
# Perform K-means clustering
kmeans = KMeans(n_clusters=2, random_state=0).fit(user_preferences)
# Predict the cluster for each user
user_clusters = kmeans.predict(user_preferences)
print("User clusters:", user_clusters)
This code uses K-means clustering to group users into clusters based on their preferences. You can then personalize content by recommending items that are popular or highly rated within each cluster.
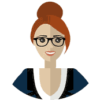
Jane Watson is a seasoned expert in AI development and a prominent author for the “Hire AI Developer” blog. With over a decade of experience in the field, Jane has established herself as a leading authority in AI app and website development, as well as AI backend integrations. Her expertise extends to managing dedicated development teams, including AI developers, Machine Learning (ML) specialists, and other supporting roles such as QA and product managers. Jane’s primary focus is on providing professional and experienced English-speaking AI developers to companies in the USA, Canada, and the UK.
Jane’s journey with AI began during her time at Duke University, where she pursued her studies in computer science. Her passion for AI grew exponentially as she delved into the intricacies of the subject. Over the years, she honed her skills and gained invaluable experience working with renowned companies such as Activision and the NSA. These experiences allowed her to master the art of integrating existing systems with AI APIs, solidifying her reputation as a versatile and resourceful AI professional.
Currently residing in the vibrant city of Los Angeles, Jane finds solace in her role as an author and developer. Outside of her professional pursuits, she cherishes the time spent with her two daughters, exploring the beautiful hills surrounding the city. Jane’s dedication to the advancement of AI technology, combined with her wealth of knowledge and experience, makes her an invaluable asset to the “Hire AI Developer” team and a trusted resource for readers seeking insights into the world of AI.