
How to Add AI to Your Website: Transform user experience, automate customer service, and personalize content with these simple integration strategies.
Integrating AI in websites has become increasingly important in web development. Adding AI to web design not only enhances user experience but also improves business productivity.
AI Integration in Web Development
Integrating AI in websites is becoming increasingly popular, with businesses looking to enhance user experience and gain a competitive edge. In web development, AI can be used to create more personalized and interactive websites, improve search engine optimization, and automate routine tasks.
AI Programming Concepts
To integrate AI into web development, it’s important to understand key AI programming concepts and how they can be applied to enhance websites. Some of the most important concepts include machine learning, natural language processing, and computer vision.
Machine learning involves teaching machines to learn from data, rather than being explicitly programmed. This enables machines to make predictions or decisions based on patterns in the data, which can be applied to a range of tasks such as personalized product recommendations or chatbot responses.
Natural language processing enables machines to understand and respond to human language, which is particularly useful for chatbots or voice assistants. Computer vision involves teaching machines to interpret visual information, which can be applied to tasks such as image recognition or video analysis.
AI Frameworks
There are a number of AI frameworks that can be used to integrate AI into web development. Some of the most popular frameworks include TensorFlow, PyTorch, and Scikit-learn.
TensorFlow is an open-source platform for machine learning developed by Google. It allows developers to create and train machine learning models using a range of programming languages, including Python, C++, and JavaScript.
PyTorch is another popular machine learning framework developed by Facebook, which is known for its ease of use and flexibility. Scikit-learn is a machine learning library for Python that provides tools for data mining and analysis.
AI Coding Examples
When integrating AI into web development, it’s important to provide code examples to illustrate AI techniques or features of frameworks. For example, the following code illustrates how to train a simple linear regression model using TensorFlow:
# Import TensorFlow library
import tensorflow as tf
# Define input data
x = tf.constant([1.0, 2.0, 3.0, 4.0])
y = tf.constant([2.0, 4.0, 6.0, 8.0])
# Define model parameters
w = tf.Variable(0.0)
b = tf.Variable(0.0)
# Define loss function
loss = tf.reduce_sum(tf.square(y - (w * x + b)))
# Define optimizer
optimizer = tf.train.GradientDescentOptimizer(0.01)
train = optimizer.minimize(loss)
# Initialize variables and run model
init = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init)
for i in range(1000):
sess.run(train)
print(sess.run([w, b]))
The above code creates a linear regression model using TensorFlow, which predicts the output (y) based on the input (x), using a linear function with parameters w and b.
The model is trained using a gradient descent optimizer to minimize the loss function, which measures the difference between the predicted output and the actual output. After running the model, the final values for w and b are printed.
Overall, understanding AI programming concepts, AI frameworks, and providing relevant AI coding examples are essential for integrating AI into web development.
By doing so, businesses can take advantage of the many benefits that AI can bring to websites and improve user experience and productivity.
Identifying Opportunities for AI in Web Design
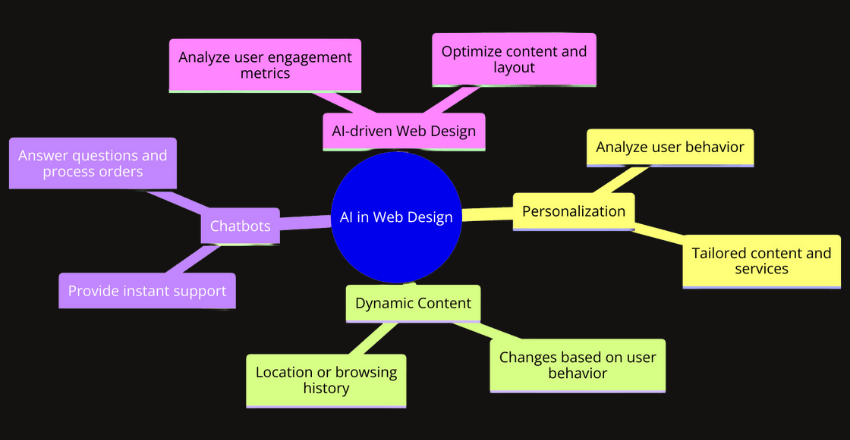
AI-driven web design is the future of website development. By using AI, websites can be tailored to the specific preferences of individual users, enhancing their experience and encouraging them to stay longer on the site. Here are some of the most promising opportunities for using AI in web design:
- Personalization: AI algorithms can analyze user behavior and preferences to offer tailored content, products, and services. This creates a more personalized experience that is likely to keep users engaged and encourage them to return to the site.
- Dynamic content: AI can be used to create dynamic content that changes based on user behavior or other factors. For example, a website may automatically show different products based on the user’s location or browsing history.
- Chatbots: AI-powered chatbots can be used to provide instant support to website visitors. They can answer questions, suggest products, and even process orders.
AI-driven Web Design
AI-driven web design involves using machine learning algorithms to analyze user behavior and preferences, and automatically adjust the website content and layout to optimize the user experience. This can include factors such as color schemes, font sizes, and layout.
By analyzing user engagement metrics such as click-through rates and time spent on site, AI can identify the most effective design elements to maximize engagement and conversion rates.
Website Personalization with AI
AI can be used to create personalized website experiences that cater to individual user preferences. By analyzing user data such as search history, purchase behavior, and preferences, AI algorithms can recommend products or content that are most relevant to the user.
This not only enhances the user experience but also increases the chances of conversion and loyalty.
By leveraging AI in web design, businesses can create highly engaging websites that provide a personalized experience to users. This not only improves user satisfaction but also contributes to increased conversions and revenue.
AI Strategies for Website Enhancement
Adding AI to website development can enhance user experience and increase business productivity. By leveraging AI strategies, websites can engage with users in real-time and guide them through their journey. Here are some of the most effective AI strategies for improving websites:
Chatbots: Incorporating chatbots can enhance customer service and improve website experience. Chatbots can provide real-time assistance, answer frequently asked questions, and guide users towards specific products or services. Take a look at this Python code example for building a simple chatbot:
from chatterbot import ChatBot
from chatterbot.trainers import ListTrainer
bot = ChatBot('MyBot')
conv = ['Hi',
'Hello',
'How are you?',
'I am good.',
'That is good to hear.',
'What are you doing?',
'I am chatting with you.']
bot.set_trainer(ListTrainer)
bot.train(conv)
while True:
request = input('You: ')
response = bot.get_response(request)
print('Bot: ', response)
Recommendation engines: Implementing recommendation engines can personalize the user experience and increase engagement. By analyzing user data and behavior, recommendation engines can suggest relevant products, services, or content based on user preferences. This is an example of how to build a recommendation engine using Python:
import pandas as pd
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
df = pd.read_csv('product_data.csv') # product data
tfidf = TfidfVectorizer(stop_words='english')
tfidf_matrix = tfidf.fit_transform(df['description'])
cosine_sim = cosine_similarity(tfidf_matrix)
indices = pd.Series(df.index, index=df['product_name']).drop_duplicates()
def recommend_products(product_name, cosine_sim=cosine_sim):
idx = indices[product_name]
sim_scores = list(enumerate(cosine_sim[idx]))
sim_scores = sorted(sim_scores, key=lambda x: x[1], reverse=True)
sim_scores = sim_scores[1:11]
product_indices = [i[0] for i in sim_scores]
return df['product_name'].iloc[product_indices]
Predictive analytics: Applying predictive analytics can help websites anticipate user behavior and deliver personalized content. By analyzing user data, predictive analytics can help websites improve their conversion rates and increase revenue. Here is an example of how to build a predictive model using R:
library(caret)
library(randomForest)
data
trainIndex
training
testing
model
predictions
confusionMatrix(predictions, testing$purchase)
By leveraging these AI strategies, websites can engage with users in real-time and guide them through their journey. However, organizations must be careful to address challenges such as data privacy, model biases, and technical limitations when implementing AI. With the right approach, AI can enhance website experience and drive business success.
AI in Web Programming: Best Practices
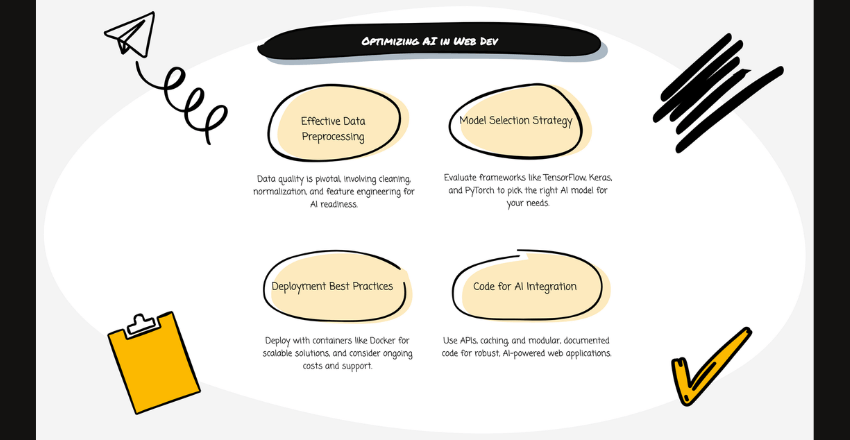
When incorporating AI into web programming, it’s important to follow certain best practices for optimal results. Here are some key considerations:
Data Preprocessing
The quality and quantity of data are essential for successful AI integration in web development. Prior to model training, data preprocessing techniques such as cleaning, normalization, and feature engineering should be used to ensure that the data is accurate and relevant.
Model Selection
Choosing the appropriate AI model for the task at hand is crucial. There are many popular AI frameworks such as TensorFlow, Keras, and PyTorch, each with their own unique features and functionalities. Code examples for each framework are readily available, making it easier to compare and select the most suitable option.
Deployment Considerations
Once the AI model has been trained, it needs to be deployed onto the website. A containerized environment such as Docker can be used for easy deployment and scaling. It’s also important to consider deployment costs and maintenance requirements.
Coding Techniques
When coding websites with AI, there are several techniques to keep in mind. For example, using RESTful APIs to connect the AI model with the website’s backend, or implementing caching mechanisms to improve performance. Additionally, keeping code modular and well-documented can make it easier to maintain and update in the future.
By following these best practices, developers can ensure that the integration of AI into web programming is successful and yields the desired results.
Overcoming Challenges in AI Integration
Despite the many benefits of integrating AI into websites, there are also several challenges that must be addressed. One major challenge is ensuring data privacy, as websites that collect and process user data must comply with various regulations and laws.
Another challenge is dealing with model biases, which can occur when the data used to train an AI system is not diverse enough. This can lead to inaccurate or unfair results, particularly in areas such as hiring or lending.
Technical limitations can also pose challenges to AI integration, as some systems may not be compatible with certain programming languages or frameworks. Additionally, AI models can be complex and resource-intensive, requiring dedicated hardware or cloud services for deployment.
To address these challenges, it is important to prioritize transparency and accountability in AI development. This includes regularly auditing and testing AI systems to ensure they are fair and unbiased. It also involves careful consideration of data privacy and security measures, as well as selecting the most appropriate AI frameworks and tools for the task at hand.
Ultimately, overcoming these challenges requires a collaborative effort between developers, businesses, and regulatory bodies to ensure that AI is developed and deployed responsibly and ethically.
AI Implementation on Different Website Types
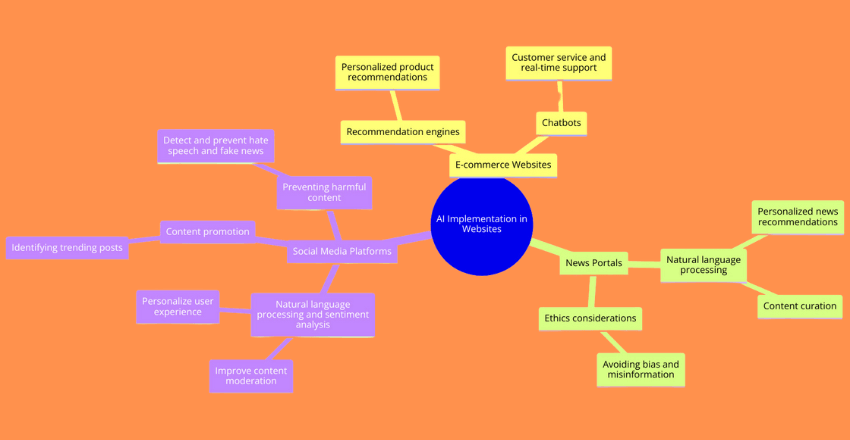
Implementing AI in a website requires a tailored approach to suit the specific needs of different website types. Whether it’s an e-commerce website, news portal or social media platform, there are certain considerations to keep in mind when integrating AI functionalities.
E-commerce Websites
E-commerce websites can benefit from AI technologies such as recommendation engines and chatbots. Recommendation engines can provide personalized product recommendations based on the user’s browsing and purchase history, while chatbots can assist in customer service and provide real-time support.
When implementing AI in e-commerce sites, it’s essential to ensure that the AI system is trained on relevant data and is capable of processing a large volume of customer interactions without compromising speed or accuracy.
News Portals
News portals can use AI functionalities such as natural language processing to provide personalized news recommendations and summaries. AI can also assist in content curation by identifying trends and relevant topics to feature on the website.
When integrating AI into news portals, it’s important to consider the ethics of using AI to curate news stories. Bias in the AI models used for news curation can lead to the promotion of certain viewpoints over others, potentially leading to misinformation and loss of credibility.
Social Media Platforms
Social media platforms can leverage AI technologies such as natural language processing and sentiment analysis to improve content moderation and personalize user experience. AI can also assist in content promotion by identifying trending posts and suggesting them to users.
When incorporating AI into social media platforms, it’s crucial to ensure that the AI system is able to detect and prevent hate speech, fake news and other forms of harmful content. Additionally, it’s important to ensure that the AI recommendations are not creating filter bubbles, where users only see content that aligns with their existing beliefs.
Wrapping up
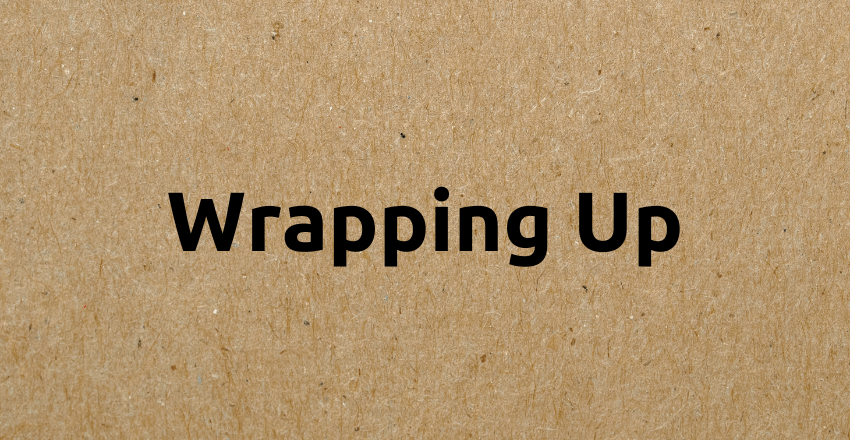
The benefits of adding AI to websites far outweigh any challenges that may arise. With a solid understanding of AI integration in web development and practical strategies for implementation, website owners can create an online presence that is both user-friendly and efficient. To stay ahead of the curve, website owners should start exploring AI integration in their web development process today.
External Resources
FAQ

FAQ 1: How can I integrate an AI chatbot into my website?
Answer:
Integrating an AI chatbot involves selecting a chatbot service and embedding it into your website’s HTML. Many platforms like Dialogflow or Microsoft Bot Framework offer easy integration options. Here’s an example using a generic chatbot platform’s embed code:
<!-- Place this code where you want the chatbot to appear on your page -->
<div id="chatbot-container"></div>
<script src="https://yourchatbotservice.com/embed.js"></script>
<script>
YourChatbotService.init({
containerId: 'chatbot-container',
apiKey: 'YOUR_API_KEY_HERE',
options: { /* Customization options */ }
});
</script>
Replace https://yourchatbotservice.com/embed.js with the actual URL provided by your chatbot service and YOUR_API_KEY_HERE with your actual API key. This script initializes the chatbot within a specified container on your page.
FAQ 2: How do I use AI to personalize website content for visitors?
Answer:
To personalize content using AI, you can implement machine learning algorithms that analyze user behavior and adjust content accordingly. Here’s a basic example using JavaScript to change content based on the time of the day:
function personalizeContent() {
const hour = new Date().getHours();
const greeting = document.getElementById('personalized-greeting');
if (hour < 12) {
greeting.innerText = 'Good morning!';
} else if (hour < 18) {
greeting.innerText = 'Good afternoon!';
} else {
greeting.innerText = 'Good evening!';
}
}
personalizeContent();
This script can be the starting point for more complex personalization, such as displaying different content based on user’s past interactions or preferences.
FAQ 3: How can I implement AI-driven search on my website?
Answer:
Implementing AI-driven search on your website can dramatically improve user experience, allowing for more natural language queries and relevant results. Here’s a simple example of integrating an AI-powered search API using JavaScript:
async function aiSearch(query) {
const response = await fetch('https://youraisearchservice.com/api/search', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_API_TOKEN'
},
body: JSON.stringify({query: query})
});
const data = await response.json();
console.log(data); // Process and display search results
}
// Example search query
aiSearch("Find articles on AI integration");
Replace https://youraisearchservice.com/api/search
and YOUR_API_TOKEN
with your AI search service’s endpoint and API token, respectively. This function sends a search query to the AI search API and logs the results, which you can then display on your website.
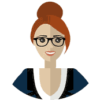
Jane Watson is a seasoned expert in AI development and a prominent author for the “Hire AI Developer” blog. With over a decade of experience in the field, Jane has established herself as a leading authority in AI app and website development, as well as AI backend integrations. Her expertise extends to managing dedicated development teams, including AI developers, Machine Learning (ML) specialists, and other supporting roles such as QA and product managers. Jane’s primary focus is on providing professional and experienced English-speaking AI developers to companies in the USA, Canada, and the UK.
Jane’s journey with AI began during her time at Duke University, where she pursued her studies in computer science. Her passion for AI grew exponentially as she delved into the intricacies of the subject. Over the years, she honed her skills and gained invaluable experience working with renowned companies such as Activision and the NSA. These experiences allowed her to master the art of integrating existing systems with AI APIs, solidifying her reputation as a versatile and resourceful AI professional.
Currently residing in the vibrant city of Los Angeles, Jane finds solace in her role as an author and developer. Outside of her professional pursuits, she cherishes the time spent with her two daughters, exploring the beautiful hills surrounding the city. Jane’s dedication to the advancement of AI technology, combined with her wealth of knowledge and experience, makes her an invaluable asset to the “Hire AI Developer” team and a trusted resource for readers seeking insights into the world of AI.